How to Create Fake Data with Faker
- prakrity rathore
- Sep 22, 2020
- 1 min read
Motivation
Let’s say you want to create data with certain data types (bool, float, text, integers) with special characteristics (names, address, color, email, phone number, location) to test some Python libraries or specific implementation. But it takes time to find that specific kind of data. You wonder: is there a quick way that you can create your own data? What if there is a package that enables you to create fake data in one line of code such as this:
fake.profile()
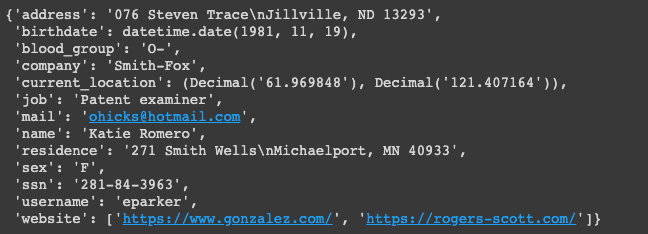
This can be done with Faker, a Python package that generates fake data for you, ranging from a specific data type to specific characteristics of that data, and the origin or language of the data. Let’s discover how we can use Faker to create fake data.
Basics of Faker
pip install Faker
from faker import Faker fake = Faker()
Some basic methods of faker:
>>> fake.color_name() 'SeaGreen' >>> fake.name() 'Vanessa Schroeder' >>> fake.address() '3138 Jennings Shore\nPort Anthony, MT 90833' >>> fake.job() 'Buyer, industrial' >>> fake.date_of_birth(minimum_age=30) datetime.date(1906, 9, 18) >>> fake.city() 'Rebeccastad'
Let’s say you are an author of a fiction book who want to create a character but find it difficult and time-consuming to come up with a realistic name and information. You can write
>>> name = fake.name() >>> color = fake.color_name() >>> city = fake.city() >>> job = fake.job() >>> print('Her name is {}. She lives in {}. Her favorite color is {}. She works as a {}'.format(name, city,color, job))
Outcome:
Her name is Natalie Gamble. She lives in East Tammyborough. Her favorite color is Magenta. She works as a Metallurgist.
With Faker, you can generate a persuasive example instantly!
Comments